How Builders Can Make improvements to Their Debugging Capabilities By Gustavo Woltmann
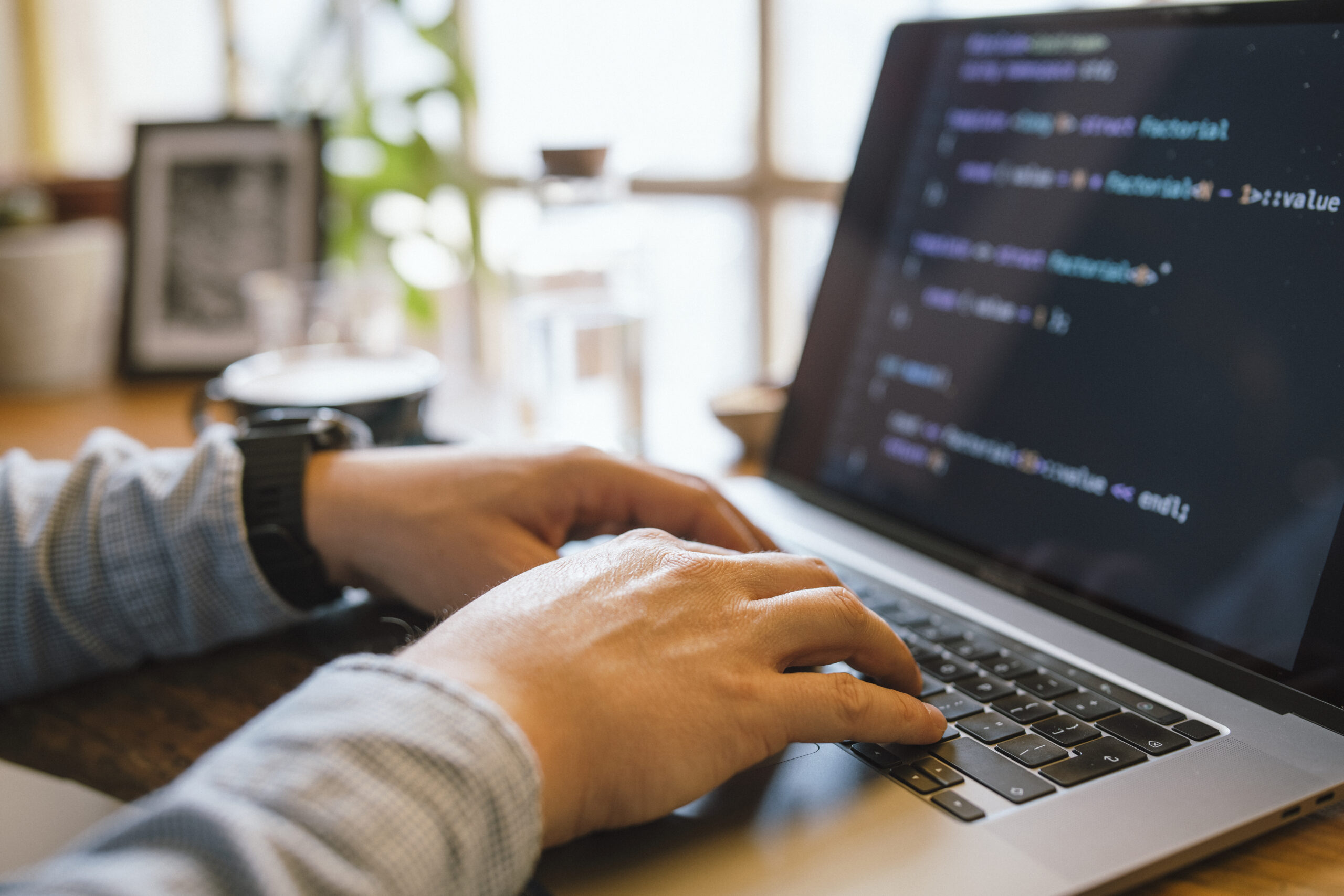
Debugging is Among the most essential — but typically missed — abilities within a developer’s toolkit. It is not almost repairing damaged code; it’s about knowledge how and why matters go wrong, and learning to Believe methodically to solve issues effectively. Regardless of whether you're a novice or even a seasoned developer, sharpening your debugging capabilities can preserve hrs of disappointment and substantially increase your productiveness. Listed below are numerous methods to assist developers amount up their debugging video game by me, Gustavo Woltmann.
Grasp Your Equipment
One of many quickest ways builders can elevate their debugging capabilities is by mastering the equipment they use daily. Whilst writing code is a person Component of growth, realizing how to connect with it properly in the course of execution is equally significant. Present day improvement environments occur Outfitted with potent debugging abilities — but several developers only scratch the floor of what these resources can perform.
Get, for example, an Built-in Improvement Ecosystem (IDE) like Visible Studio Code, IntelliJ, or Eclipse. These tools help you set breakpoints, inspect the worth of variables at runtime, stage through code line by line, and in some cases modify code around the fly. When applied appropriately, they Permit you to observe particularly how your code behaves in the course of execution, which happens to be invaluable for monitoring down elusive bugs.
Browser developer tools, for example Chrome DevTools, are indispensable for front-stop builders. They permit you to inspect the DOM, watch network requests, check out serious-time efficiency metrics, and debug JavaScript from the browser. Mastering the console, sources, and network tabs can convert irritating UI troubles into workable tasks.
For backend or program-amount builders, resources like GDB (GNU Debugger), Valgrind, or LLDB offer you deep Command more than managing procedures and memory administration. Studying these equipment could possibly have a steeper learning curve but pays off when debugging efficiency difficulties, memory leaks, or segmentation faults.
Further than your IDE or debugger, become cozy with Edition Regulate units like Git to understand code background, locate the precise minute bugs had been launched, and isolate problematic alterations.
In the long run, mastering your instruments usually means likely beyond default settings and shortcuts — it’s about creating an intimate understanding of your advancement surroundings to ensure when troubles occur, you’re not missing in the dead of night. The higher you recognize your instruments, the more time it is possible to devote fixing the actual problem rather than fumbling through the procedure.
Reproduce the condition
One of the more important — and sometimes disregarded — measures in efficient debugging is reproducing the issue. Before leaping to the code or producing guesses, developers have to have to produce a steady atmosphere or scenario where by the bug reliably seems. With out reproducibility, correcting a bug will become a match of likelihood, frequently leading to wasted time and fragile code changes.
The initial step in reproducing a challenge is collecting as much context as feasible. Question concerns like: What steps brought about The problem? Which environment was it in — enhancement, staging, or creation? Are there any logs, screenshots, or error messages? The greater detail you have got, the less complicated it gets to be to isolate the precise ailments beneath which the bug takes place.
As soon as you’ve collected ample information, endeavor to recreate the issue in your neighborhood environment. This might mean inputting precisely the same data, simulating related person interactions, or mimicking program states. If The difficulty appears intermittently, take into account crafting automated assessments that replicate the sting instances or condition transitions associated. These tests not merely assistance expose the trouble and also prevent regressions Later on.
From time to time, the issue could be natural environment-specific — it might come about only on selected operating methods, browsers, or beneath unique configurations. Using resources like virtual devices, containerization (e.g., Docker), or cross-browser screening platforms is often instrumental in replicating such bugs.
Reproducing the issue isn’t only a phase — it’s a way of thinking. It necessitates patience, observation, along with a methodical strategy. But as soon as you can persistently recreate the bug, you happen to be now midway to correcting it. Which has a reproducible state of affairs, you can use your debugging tools much more successfully, check prospective fixes securely, and talk much more clearly together with your group or customers. It turns an abstract criticism into a concrete obstacle — Which’s wherever builders thrive.
Go through and Realize the Error Messages
Mistake messages will often be the most beneficial clues a developer has when a thing goes Erroneous. As an alternative to viewing them as irritating interruptions, developers should really study to deal with error messages as immediate communications through the program. They frequently tell you what precisely took place, in which it happened, and in some cases even why it took place — if you know how to interpret them.
Get started by looking at the concept carefully As well as in total. Many builders, especially when less than time force, glance at the main line and quickly begin earning assumptions. But deeper in the error stack or logs may lie the genuine root result in. Don’t just duplicate and paste error messages into search engines — examine and comprehend them to start with.
Break the mistake down into components. Could it be a syntax error, a runtime exception, or possibly a logic mistake? Does it issue to a particular file and line range? What module or perform activated it? These concerns can tutorial your investigation and point you toward the liable code.
It’s also beneficial to be familiar with the terminology from the programming language or framework you’re working with. Error messages in languages like Python, JavaScript, or Java usually abide by predictable patterns, and Finding out to acknowledge these can significantly increase your debugging procedure.
Some problems are imprecise or generic, and in Individuals scenarios, it’s crucial to examine the context through which the mistake occurred. Verify relevant log entries, enter values, and up to date modifications inside the codebase.
Don’t forget compiler or linter warnings possibly. These often precede greater difficulties and supply hints about potential bugs.
In the end, error messages will not be your enemies—they’re your guides. Mastering to interpret them the right way turns chaos into clarity, helping you pinpoint problems more rapidly, lessen debugging time, and turn into a additional economical and self-assured developer.
Use Logging Sensibly
Logging is one of the most potent resources within a developer’s debugging toolkit. When utilised properly, it offers true-time insights into how an application behaves, supporting you recognize what’s occurring beneath the hood with no need to pause execution or stage with the code line by line.
An excellent logging method starts with understanding what to log and at what level. Common logging levels involve DEBUG, Facts, Alert, ERROR, and FATAL. Use DEBUG for detailed diagnostic information all through enhancement, Details for standard activities (like productive begin-ups), Alert for probable troubles that don’t break the applying, Mistake for true issues, and Lethal if the program can’t continue.
Stay clear of flooding your logs with abnormal or irrelevant info. An excessive amount of logging can obscure vital messages and decelerate your method. Focus on critical functions, state changes, enter/output values, and critical final decision factors in your code.
Structure your log messages clearly and continually. Contain context, which include timestamps, request IDs, and performance names, so it’s simpler to trace challenges in distributed systems or multi-threaded environments. Structured logging (e.g., JSON logs) might make it even easier to parse and filter logs programmatically.
For the duration of debugging, logs let you observe how variables evolve, what conditions are fulfilled, and what branches of logic are executed—all without halting the program. They’re especially worthwhile in output environments in which stepping as a result of code isn’t achievable.
On top of that, use logging frameworks and resources (like Log4j, Winston, or Python’s logging module) that guidance log rotation, filtering, and integration with monitoring dashboards.
In the end, clever logging is about balance and clarity. By using a perfectly-believed-out logging technique, you can reduce the time it will require to identify problems, achieve further visibility into your purposes, and improve the Total maintainability and trustworthiness of your code.
Believe Just like a Detective
Debugging is not simply a technological job—it's a kind of investigation. To proficiently identify and repair bugs, developers have to tactic the procedure like a detective solving a mystery. This attitude will help stop working advanced challenges into workable parts and adhere to clues logically to uncover the root result in.
Start off by collecting proof. Consider the signs or symptoms of the condition: mistake messages, incorrect output, or general performance issues. Just like a detective surveys a crime scene, collect just as much applicable information as you can without leaping to conclusions. Use logs, exam conditions, and person stories to piece jointly a transparent photo of what’s occurring.
Following, kind hypotheses. Request oneself: What might be creating this behavior? Have any variations a short while ago been designed to your codebase? Has this situation occurred before less than very similar conditions? The aim would be to slender down options and recognize possible culprits.
Then, test your theories systematically. Endeavor to recreate the challenge within a managed natural environment. Should you suspect a specific purpose or element, isolate it and verify if The problem persists. Like a detective conducting interviews, check with your code queries and let the final results lead you nearer to the truth.
Pay back near attention to smaller particulars. Bugs normally conceal in the minimum expected sites—just like a lacking semicolon, an off-by-a single mistake, or possibly a race condition. Be extensive and patient, resisting the urge to patch The problem without thoroughly knowing it. Non permanent fixes could disguise the real challenge, only for it to resurface later on.
Lastly, hold notes on what you experimented with and acquired. Just as detectives log their investigations, documenting your debugging course of action can conserve time for long run problems and support others recognize your reasoning.
By thinking just like a detective, builders can sharpen their analytical expertise, tactic problems methodically, and turn into more practical at uncovering concealed problems in intricate units.
Write Exams
Composing checks is among the simplest methods to boost your debugging capabilities and Over-all development efficiency. Tests not just aid catch bugs early and also function a safety net that provides you self esteem when making modifications in your codebase. A properly-examined software is simpler to debug as it means that you can pinpoint accurately where and when a problem occurs.
Start with unit tests, which concentrate on person functions or modules. These little, isolated tests can rapidly reveal whether a specific bit of logic is Doing the job as envisioned. Any time a exam fails, you promptly know wherever to glance, drastically minimizing enough time put in debugging. Unit tests are especially practical for catching regression bugs—difficulties that reappear immediately after Formerly getting set.
Next, combine integration assessments and conclude-to-stop tests into your workflow. These assistance be sure that a variety of areas of your application do the job jointly easily. They’re particularly practical for catching bugs that come about in intricate methods with various parts or providers interacting. If something breaks, your assessments can tell you which Element of the pipeline failed and under what ailments.
Composing checks also forces you to definitely Believe critically regarding your code. To test a aspect effectively, you need to be aware of its inputs, expected outputs, and edge conditions. This degree of understanding In a natural way leads to higher code composition and less bugs.
When debugging a difficulty, writing a failing examination that reproduces the bug can be a strong starting point. Once the examination fails continuously, you are able to center on fixing the bug and look at your test pass when The problem is fixed. This method makes certain that the same bug doesn’t return Later on.
Briefly, writing exams turns debugging from a frustrating guessing sport into a structured and predictable course of action—helping you catch far more bugs, a lot quicker and more reliably.
Get Breaks
When debugging a difficult challenge, it’s quick to become immersed in the issue—watching your display screen for several hours, striving Option after Remedy. But The most underrated debugging instruments is actually stepping absent. Taking breaks assists you reset your thoughts, lessen annoyance, and infrequently see The difficulty from the new standpoint.
If you're far too near the code for too lengthy, cognitive fatigue sets in. You may begin overlooking apparent mistakes or misreading code that you just wrote just hrs earlier. Within this state, your Mind results in being fewer economical at challenge-fixing. A short walk, a espresso crack, or simply switching to another undertaking for ten–15 minutes can refresh your focus. Many here builders report obtaining the basis of a problem when they've taken time and energy to disconnect, allowing their subconscious work during the qualifications.
Breaks also aid prevent burnout, Primarily through for a longer time debugging sessions. Sitting down in front of a monitor, mentally caught, is not only unproductive and also draining. Stepping away allows you to return with renewed Electrical power plus a clearer state of mind. You may perhaps out of the blue recognize a missing semicolon, a logic flaw, or maybe a misplaced variable that eluded you just before.
In the event you’re trapped, a great general guideline is always to established a timer—debug actively for 45–sixty minutes, then take a 5–ten minute split. Use that point to move all-around, stretch, or do a thing unrelated to code. It might sense counterintuitive, Specifically less than restricted deadlines, but it really in fact causes more rapidly and more practical debugging Over time.
To put it briefly, using breaks will not be a sign of weak point—it’s a smart method. It presents your Mind Area to breathe, enhances your standpoint, and assists you stay away from the tunnel eyesight that often blocks your progress. Debugging can be a psychological puzzle, and relaxation is part of fixing it.
Study From Each Bug
Each and every bug you face is a lot more than just a temporary setback—It really is a possibility to develop being a developer. Irrespective of whether it’s a syntax error, a logic flaw, or even a deep architectural situation, every one can instruct you something worthwhile when you go to the trouble to reflect and analyze what went Improper.
Start off by inquiring by yourself some vital thoughts once the bug is resolved: What caused it? Why did it go unnoticed? Could it happen to be caught earlier with much better methods like unit screening, code testimonials, or logging? The solutions typically reveal blind spots inside your workflow or knowing and enable you to Construct more powerful coding routines shifting forward.
Documenting bugs can also be an excellent pattern. Continue to keep a developer journal or retain a log in which you Notice down bugs you’ve encountered, how you solved them, and what you learned. Eventually, you’ll begin to see designs—recurring concerns or typical mistakes—you could proactively prevent.
In crew environments, sharing Everything you've learned from the bug using your peers can be Primarily strong. Regardless of whether it’s through a Slack information, a short write-up, or A fast information-sharing session, helping Some others stay away from the same challenge boosts group efficiency and cultivates a more robust Studying society.
A lot more importantly, viewing bugs as classes shifts your frame of mind from aggravation to curiosity. In place of dreading bugs, you’ll commence appreciating them as critical areas of your development journey. All things considered, a few of the finest developers are certainly not the ones who publish perfect code, but individuals who continuously understand from their issues.
Ultimately, Each individual bug you repair provides a new layer in your talent established. So up coming time you squash a bug, have a moment to reflect—you’ll appear absent a smarter, much more capable developer thanks to it.
Conclusion
Bettering your debugging techniques requires time, follow, and endurance — but the payoff is huge. It can make you a far more efficient, assured, and capable developer. The subsequent time you might be knee-deep in a mysterious bug, don't forget: debugging isn’t a chore — it’s a possibility to be better at Everything you do.